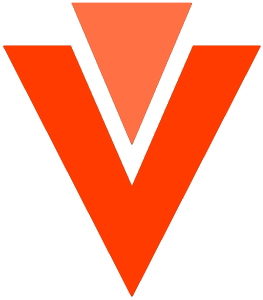
Svelteify
Material components framework for Svelte
Beautiful
The framework works with the stylesheet of Vuetify, which provides clean and nice-looking components.
Customizable
You can configure colors used across the application, and a dark mode. Moreover, you can precisely choose which component to use.
Dependencies-less
The library includes all the compiled JavaScript and CSS, so you don't have to install anything or setup additional tools.
Getting started
Base guidelines for the frameworkInstallation
The installation of the library is pretty simple. First, go into your application's folder and download Svelteify from npmjs.org :
npm install --dev svelteify # Using NPM
yarn add --dev svelteify # Using Yarn
Stylesheets
For stylesheets import, you have two options :
- From the web : import the stylesheet at
https://svelteify.exybo.re/svelteify.css
- Using a CSS loader : include the
svelteify/dist/svelteify.css
file in your code. Don't forget to add a CSS loader in your bundler, like Webpack or Rollup !
Finally, include the Roboto font and the Material Icons from Google Fonts :
https://fonts.googleapis.com/css?family=Roboto:100,300,400,500,700,900|Material+Icons
The MApp component
An MApp
component is required at the root of
your application. It registers the theme as well as some components logic. If
you don't do so, it'll not be working as expected.
Other layout components
The MToolbar
component helps you have a navigation bar at the top
of your app, and the MFooter
component to have a footer at its
end.
The MContent
component contains the main page and all of its
content. It's essential for the grid system to work properly, but not vital
for the app.
Example
Here you can see a basic layout for a starter app :
<MApp>
<MToolbar></Mtoolbar>
<MContent>
<MContainer fluid>
</MContainer>
</MContent>
<MFooter></MFooter>
</MApp>
Each element can be colored, with the color
property or not : in
fact, colors are just CSS classes.
<MBtn color="amber lighten-1 white--text">
Button
</MBtn>
Hello there !
<p class="red--text text--darken-2">Hello there !</p>
You can see a complete list of the available colors on the Vuetify documentation.
UI Components
All you need to create your appAvatar
The Avatar component is a little helper to transform images into nice looking avatars.
Properties for component MAvatar
Name | Type | Description | Default value |
---|---|---|---|
color | string | Applies a custom color (text or background) | "" |
size | string | Applies a custom size | "48px" |
tile | boolean | Makes the avatar squared | false |
Examples
Avatar

<MAvatar>
<img src="https://exybore.becauseofprog.fr/img/avatar.jpg" />
</MAvatar>
Custom-sized avatar

<MAvatar size="75px">
<img src="https://exybore.becauseofprog.fr/img/avatar.jpg" />
</MAvatar>
Tile avatar

<MAvatar tile>
<img src="https://exybore.becauseofprog.fr/img/avatar.jpg" />
</MAvatar>
Button
Buttons are essential in an app : they indicate to user where it can click to do some actions.
Properties for component MBtn
Name | Type | Description | Default value |
---|---|---|---|
absolute | boolean | Applies an absolute position to the component | false |
block | boolean | Makes the button full width | false |
bottom | boolean | Puts the button at the bottom | false |
classes | string | Applies CSS classes to the button | "" |
color | string | Applies a custom color to the button (text or background) | "" |
dark | boolean | Applies a dark theme to the button | false |
depressed | boolean | Removes the button box shadow | false |
disabled | boolean | Disables the button so it's not clickable | false |
fixed | boolean | Applies a fixed position to the button | false |
flat | boolean | Removes the button background color | false |
floating | boolean | Transforms the button into a floating-action button | false |
href | string | Sets the link associated to the button | "#!" |
target | string | Sets link's target | "" |
icon | boolean | Makes an icon button | false |
large | boolean | Sets button's size to large | false |
left | boolean | Puts the button to the left | false |
light | boolean | Applies a light theme to the button | false |
outline | boolean | Keeps only a border around the button | false |
right | boolean | Puts the button at the right | false |
round | boolean | Makes the button round | false |
small | boolean | Sets button's size to small | false |
top | boolean | Puts the button to the top | false |
Examples
Button
<MBtn>Button</MBtn>
Colored button
<MBtn color="pink white--text">Button</MBtn>
Outline button
<MBtn outline color="green">Button</MBtn>
Flat button
<MBtn flat>Button</MBtn>
Round button
<MBtn round>Button</MBtn>
Block button
<MBtn block>Button</MBtn>
Small button
<MBtn small>Button</MBtn>
Large button
<MBtn large>Button</MBtn>
Floating action button
<MBtn floating icon color="red">
<MIcon>edit</MIcon>
</MBtn>
Card
Cards are panels which display information and sometimes images. They're also useful to highlight parts of the content.
Properties for component MCard
Name | Type | Description | Default value |
---|---|---|---|
color | string | Applies a custom color (text or background) | "" |
dark | boolean | Applies a dark theme to the card | false |
elevation | integer | Specifies a custom elevation of the card | 2 |
flat | boolean | Removes the shadow of the card | false |
hover | boolean | Applies an additional shadow effect on hover | false |
light | boolean | Applies a light theme to the card | false |
raised | boolean | Adds a higher default elevation | false |
tile | boolean | Makes the card squared | false |
Properties for component MCardTitle
Name | Type | Description | Default value |
---|---|---|---|
primary_title | boolean | Specifies if the title is primary | false |
Examples
Classic card
Kangaroo Valley Safari
<MFlex size="xs12 sm6 md3">
<MCard>
<MImg
src="https://cdn.vuetifyjs.com/images/cards/desert.jpg"
alt="Background"
/>
<MCardTitle primary_title>
<div>
<h3 class="headline mb-0">Kangaroo Valley Safari</h3>
<div>
Lorem ipsum dolor sit amet, brute iriure accusata ne mea. Eos
suavitate referrentur ad, te duo agam libris qualisque, utroque
quaestio accommodare no qui. Et percipit laboramus usu, no invidunt
verterem nominati mel. Dolorem ancillae an mei, ut putant invenire
splendide mel, ea nec propriae adipisci. Ignota salutandi accusamus in
sed, et per malis fuisset, qui id ludus appareat.
</div>
</div>
</MCardTitle>
<MCardActions>
<MBtn flat color="orange--text">Share</MBtn>
<MBtn flat color="orange--text">Explore</MBtn>
</MCardActions>
</MCard>
</MFlex>
Image card
<MFlex size="xs12 sm6 md3">
<MCard>
<MImg
src="https://exybore.becauseofprog.fr/img/background.jpg"
alt="Background"
/>
<MCardActions>
<MBtn flat icon color="orange--text">
<MIcon>favorite</MIcon>
</MBtn>
<MBtn flat icon color="orange--text">
<MIcon>bookmark</MIcon>
</MBtn>
<MBtn flat icon color="orange--text">
<MIcon>share</MIcon>
</MBtn>
</MCardActions>
</MCard>
</MFlex>
Chip
Chips are piece of information contained in a little box.
Properties for component MChip
Name | Type | Description | Default value |
---|---|---|---|
close | boolean | Adds a button to close the chip | false |
color | string | Applies a custom color (text or background) | "" |
dark | boolean | Applies a dark theme to the chip | false |
disabled | boolean | Disables the chip so it can't be clicked | false |
label | boolean | Transforms the chip into a little label | false |
light | boolean | Applies a light theme to the chip | false |
outline | boolean | Keeps only a border around the chip | false |
selected | boolean | Checks if the chip is selected | false |
small | boolean | Sets chip's size to small | false |
Examples
Chip
<MChip>
Example chip
</MChip>
Colored chip
<MChip color="green white--text">
Example chip
</MChip>
Themed chip
<MChip color="primary">
Example chip
</MChip>
Outline chip
<MChip outline color="purple purple--text">
Example chips
</MChip>
Small chip
<MChip small>
Example chips
</MChip>
Closable chip
<MChip close>
Example chips
</MChip>
Avatar chip

<MChip>
<MAvatar>
<img src="https://exybore.becauseofprog.fr/img/avatar.jpg" />
</MAvatar>
Example chip
</MChip>
Icon chip
<MChip>
<MAvatar>
<MIcon>edit</MIcon>
</MAvatar>
Example chip
</MChip>
Icon
Icons are glyphs that can be placed across the application to add details.
Properties for component MIcon
Name | Type | Description | Default value |
---|---|---|---|
color | string | Applies a custom color (text or background) | "" |
dark | boolean | Applies a dark theme to the icon | false |
large | boolean | Sets icon's size to large | false |
left | boolean | Places the icon at the left | false |
light | boolean | Applies a light theme to the icon | false |
medium | boolean | Sets icon's size to medium | false |
right | boolean | Places the icon at the right | false |
small | boolean | Sets icon's size to small | false |
size | integer | Applies a custom size for the icon | undefined |
xlarge | boolean | Sets icon's size to xlarge | false |
Examples
Check out the Material Icons page for a full list of icons.Icon sizes
You can set the icon size of your choice according to your needs.
<MLayout row>
<MIcon small>home</MIcon>
<MIcon small>event</MIcon>
<MIcon small>info</MIcon>
</MLayout>
<MLayout row>
<MIcon>home</MIcon>
<MIcon>event</MIcon>
<MIcon>info</MIcon>
</MLayout>
<MLayout row>
<MIcon medium>home</MIcon>
<MIcon medium>event</MIcon>
<MIcon medium>info</MIcon>
</MLayout>
<MLayout row>
<MIcon large>home</MIcon>
<MIcon large>event</MIcon>
<MIcon large>info</MIcon>
</MLayout>
<MLayout row>
<MIcon xlarge>home</MIcon>
<MIcon xlarge>event</MIcon>
<MIcon xlarge>info</MIcon>
</MLayout>
Colored icons
The icons are colorable using the helpers. If you want to color the icon itself, use the text mode.
<MLayout row>
<MIcon color="green--text">home</MIcon>
<MIcon color="amber--text">event</MIcon>
<MIcon color="light-blue--text">info</MIcon>
</MLayout>
Toolbar
A toolbar is generally the primary source of site navigation and placed at the very top of the page.
Properties for component MToolbar
Name | Type | Description | Default value |
---|---|---|---|
absolute | boolean | Applies an absolute position to the toolbar | false |
card | boolean | Inherits corner rounding when used within card | false |
classes | string | Applies CSS classes to the toolbar | "" |
color | string | Applies a custom color (text or background) | "" |
dark | boolean | Applies a dark theme to the toolbar | false |
dense | boolean | Reduces the height of the toolbar | false |
extended | boolean | Extends the height of the toolbar | false |
fixed | boolean | Fixes the toolbar at the top of the page | false |
flat | boolean | Removes the shadow of the toolbar | false |
floating | boolean | Applies a floating position to the toolbar | false |
light | boolean | Applies a light theme to the toolbar | false |
tabs | boolean | Adds an extra space for tabs | false |
Properties for component MToolbarTitle
Name | Type | Description | Default value |
---|---|---|---|
classes | string | Applies CSS classes to the toolbar | "" |
Properties for component MToolbarSideIcon
Name | Type | Description | Default value |
---|---|---|---|
dark | boolean | Applies a dark theme to the toolbar | false |
Properties for component MToolbarItems
Name | Type | Description | Default value |
---|---|---|---|
classes | string | Applies CSS classes to the toolbar | "" |
Examples
Classic toolbar
<MToolbar>
<MToolbarSideIcon />
<MToolbarTitle>Toolbar</MToolbarTitle>
<div class="spacer" />
<MToolbarItems>
<MBtn flat>Link</MBtn>
<MBtn flat>Link</MBtn>
<MBtn flat>Link</MBtn>
</MToolbarItems>
</MToolbar>
Colored toolbar
<MToolbar color="green">
<MToolbarSideIcon dark />
<MToolbarTitle classes="white--text">Toolbar</MToolbarTitle>
<div class="spacer" />
<MToolbarItems>
<MBtn flat color="white--text">Link</MBtn>
<MBtn flat color="white--text">Link</MBtn>
<MBtn flat color="white--text">Link</MBtn>
</MToolbarItems>
</MToolbar>
Themed toolbar
<MToolbar color="primary">
<MToolbarSideIcon dark />
<MToolbarTitle classes="white--text">Toolbar</MToolbarTitle>
<div class="spacer" />
<MToolbarItems>
<MBtn flat color="white--text">Link</MBtn>
<MBtn flat color="white--text">Link</MBtn>
<MBtn flat color="white--text">Link</MBtn>
</MToolbarItems>
</MToolbar>
Dense toolbar
<MToolbar dense>
<MToolbarSideIcon />
<MToolbarTitle>Toolbar</MToolbarTitle>
<div class="spacer" />
<MToolbarItems>
<MBtn flat>Link</MBtn>
<MBtn flat>Link</MBtn>
<MBtn flat>Link</MBtn>
</MToolbarItems>
</MToolbar>
Extended toolbar
<MToolbar extended>
<MToolbarSideIcon />
<MToolbarTitle>Toolbar</MToolbarTitle>
<div class="spacer" />
<MToolbarItems>
<MBtn flat>Link</MBtn>
<MBtn flat>Link</MBtn>
<MBtn flat>Link</MBtn>
</MToolbarItems>
</MToolbar>
Toolbar with dividers
<MToolbar>
<MToolbarSideIcon />
<MToolbarTitle>Toolbar</MToolbarTitle>
<div class="spacer" />
<MToolbarItems>
<MBtn flat>Link 1</MBtn>
<MDivider vertical classes="mx-2" />
<MBtn flat>Link 2</MBtn>
</MToolbarItems>
</MToolbar>
Search bar
<MToolbar dense>
<div class="v-input v-text-field v-text-field--single-line">
<div class="v-input__prepend-outer">
<div class="v-input__icon--prepend">
<MIcon>search</MIcon>
</div>
</div>
<div class="v-input__control">
<div class="v-input__slot">
<div class="v-text-field__slot">
<input type="text" />
</div>
</div>
</div>
</div>
<MBtn icon>
<MIcon>my_location</MIcon>
</MBtn>
<MBtn icon>
<MIcon>more_vert</MIcon>
</MBtn>
</MToolbar>